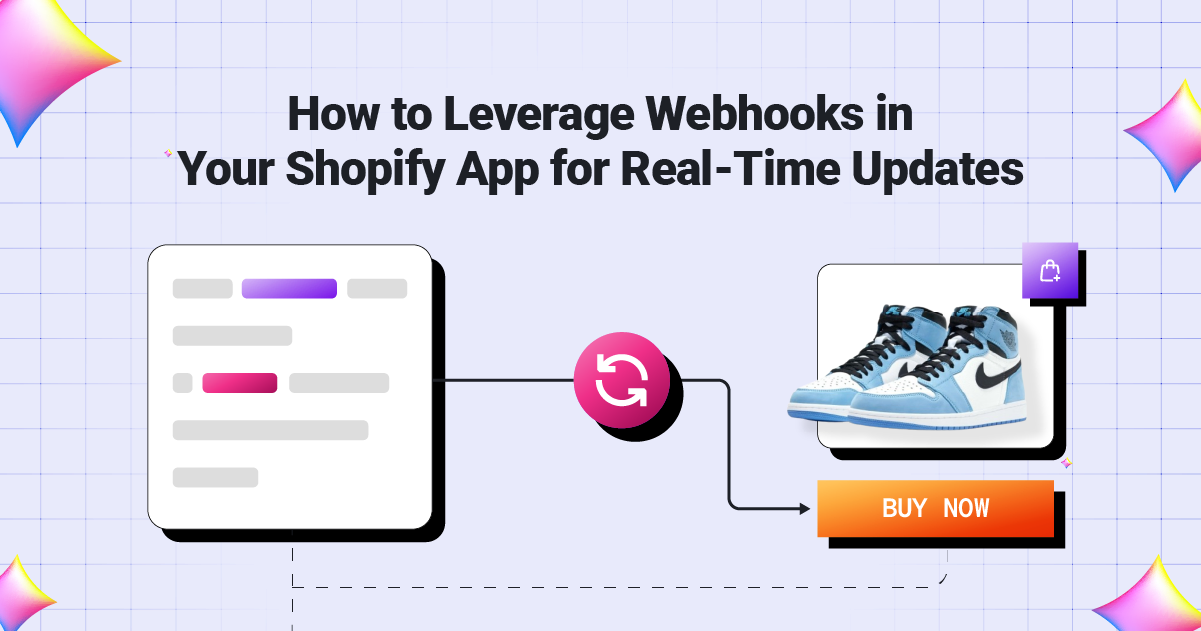
In today’s fast-paced eCommerce world, real-time updates are crucial for delivering a seamless user experience. If you’re building a Shopify app, webhooks offer an efficient way to receive instant notifications about store events. Whether you want to track order updates, customer actions, or inventory changes, webhooks can automate workflows and reduce reliance on manual data fetching.
This guide will explain how to leverage Shopify webhooks for real-time updates, ensuring your app functions smoothly and enhances store performance.
What Are Shopify Webhooks?
Webhooks are automated messages sent from Shopify to an external server when a specific event occurs in a store. Unlike APIs that require periodic polling for data, webhooks push information instantly, making them more efficient.
Benefits of Using Webhooks:
- Real-Time Data: Get instant updates without making frequent API requests.
- Efficiency: Reduce server load and bandwidth usage.
- Automation: Trigger workflows automatically based on store events.
- Improved User Experience: Ensure timely order processing and customer notifications.
Setting Up Webhooks in Shopify
Setting up webhooks in Shopify is straightforward. Follow these steps to integrate them into your app:
Step 1: Choose the Event
First, determine the event you want to track. Shopify provides webhooks for various store activities, such as:
- Orders (creation, updates, fulfillment, cancellation)
- Products (creation, updates, deletion)
- Customers (creation, updates, deletion)
Step 2: Register a Webhook in Shopify
You can create a webhook using Shopify’s admin panel or API.
Using Shopify Admin:
- Go to Settings > Notifications in your Shopify dashboard.
- Scroll down to the Webhooks section.
- Click Create a webhook and select the event type.
- Enter your server URL where Shopify will send data.
- Choose the format (JSON or XML) and save the webhook.
Using Shopify API:
If you’re developing an app, you can register a webhook using the Shopify API:
POST /admin/api/2023-01/webhooks.json
{
“webhook”: {
“topic”: “orders/create”,
“address”: “https://your-server.com/webhook-handler”,
“format”: “json”
}
}
Step 3: Handle Webhook Data
Once the webhook is set up, Shopify will send a payload to your specified URL whenever the event occurs. Your server must process this data efficiently.
Example code in Node.js:
const express = require(“express”);
const bodyParser = require(“body-parser”);
const app = express();
app.use(bodyParser.json());
app.post(“/webhook-handler”, (req, res) => {
console.log(“Webhook received:”, req.body);
res.sendStatus(200);
});
app.listen(3000, () => console.log(“Server running on port 3000”));
Step 4: Secure Your Webhook
Shopify includes an HMAC (Hash-Based Message Authentication Code) in webhook requests to verify authenticity. You should validate this HMAC to prevent unauthorized access.
Example verification in Node.js:
const crypto = require(“crypto”);
function verifyWebhook(req, res, next) {
const hmac = req.headers[“x-shopify-hmac-sha256”];
const body = JSON.stringify(req.body);
const secret = “your-secret-key”;
const hash = crypto.createHmac(“sha256”, secret).update(body).digest(“base64”);
if (hash === hmac) {
next();
} else {
res.status(401).send(“Unauthorized”);
}
}
Best Practices for Using Webhooks in Your Shopify App
1. Use Webhook Retries
Shopify retries webhook delivery up to 19 times over 48 hours if the response is not received. Ensure your server can handle failures and retry logic.
2. Optimize Processing Time
Webhook requests should be processed quickly. Offload heavy tasks to background jobs to prevent timeouts.
3. Monitor Webhook Logs
Regularly check logs to ensure webhooks are firing correctly. Use error monitoring tools to detect failures.
4. Keep Your Webhooks Updated
Shopify may introduce new webhook versions. Always use the latest API version to avoid deprecated events.
Conclusion
Webhooks are a powerful tool for enhancing your Shopify app’s functionality by providing real-time updates. By setting up webhooks correctly, handling data securely, and following best practices, you can optimize automation and improve user experience.
If you’re looking for a Shopify development company to build or enhance your Shopify store with advanced features like webhooks, contact Exinent today.